A very first step to enter into coding practice
Today we will be discussing about arrays .
ARRAYS: It is a linear data structure used to store a list of homogeneous data under a single name.
Consider a program in which you need to store 10 values and add 10 other values to that then in that case you need to define 20 different variable names instead of all that mess we can uses arrays
ARRAYS IN C:
ARRAY REPRESENTATION
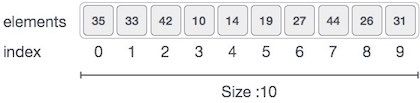
As you can see here data is stored in A and each element can be referenced using index value.
suppose a[0] is accessed it's value is 35
same as a[1]=33 ,a[2]=42 and so on.
Array indexes always start with zero because a[0] is base address of an array and a[1] can be accessed as a[0+1] where '1' is index
in the same way a[2]=a[0+2] and so on
ACCESS AN ARRAY IN PROGRAM
A FOR loop will be a very convenient way to access an array
even while, do-while can also be used.
A common for loop looks like
for(initialization;condition;value_change)
we all know how to use a increment loop .Below is implementation of for loop which decrements.
for(int i=range-1;i>=0;i--) DECREMENT for LOOP
ASSIGNMENT IN C:
If value in an array is to be initialized in c , assignment operator can be used
For example a[0] need to be initialized to 100
then syntax looks like a[0]=100;
ARRAYS IN PYTHON:
LISTS can be considered as arrays in python.
Unlike C ,Python doesn't require any declaration part.
a=[ ] "creates a new list with name a"
A very good advantage of python is dynamic length change,it can store heterogeneous types of data.
Example of lists in python are
a=['1' ,1,'string','character']
Functions in python for list manipulation:
APPEND:
Adds data into a list,
syntax:
list_name.append("data to be appended")
a=[ ]
a.append(1)
a.append(2)
print(a)
a value is [ 1 ,1 ]
EXTEND:
Appends a list to other
syntax:
list_name_1.extend(list_name_2)
A=[1,2 ,3 ]
B=[2,3,6 ]
A.extend(B)
print(A)
A becomes [ 1,2,3,2,3,6 ]
SORT:
sorts data in a list
syntax:
list_name.sort()
it sorts a list in ascending order.
A=[4,2,9,0 ]
A.sort()
print(A)
A is now equal to [ 0,2,4,9 ]
sort can be done even in a reverse order.
list_name.sort(reverse=True)
A=[4,2,9,0 ]
A.sort(reverse=True)
print(A)
A is [9,4,2,0]
To be continued..
Thank you for reading.